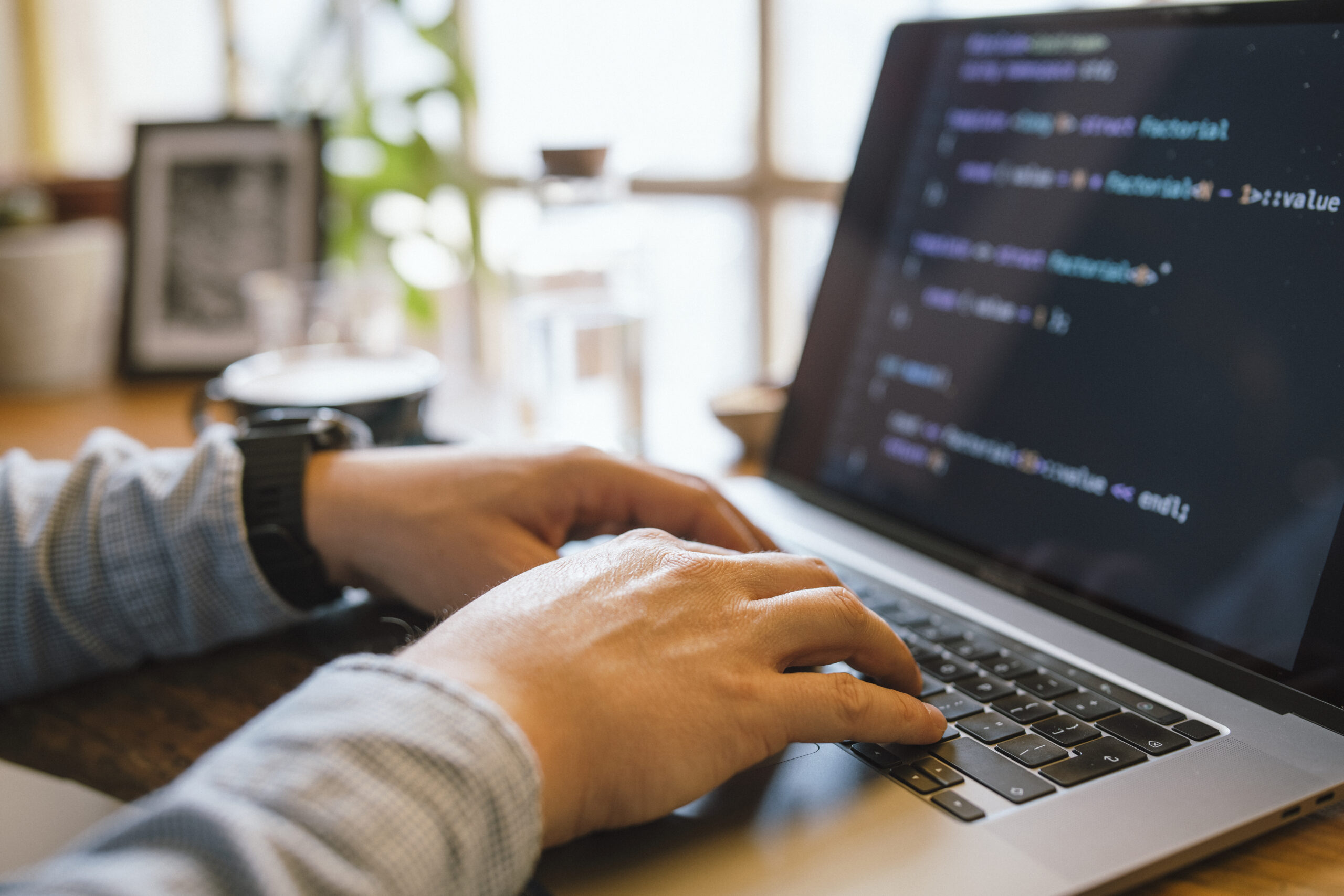
Debugging is The most crucial — still often disregarded — techniques inside of a developer’s toolkit. It's not nearly repairing damaged code; it’s about understanding how and why matters go wrong, and learning to think methodically to solve issues effectively. No matter whether you're a novice or possibly a seasoned developer, sharpening your debugging capabilities can preserve hrs of stress and substantially enhance your productivity. Here are numerous approaches that will help builders stage up their debugging match by me, Gustavo Woltmann.
Learn Your Applications
Among the list of fastest means builders can elevate their debugging techniques is by mastering the instruments they use on a daily basis. When crafting code is a person Element of progress, being aware of the best way to interact with it effectively in the course of execution is equally essential. Modern progress environments appear equipped with impressive debugging capabilities — but numerous builders only scratch the surface area of what these tools can perform.
Take, such as, an Integrated Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, move by way of code line by line, as well as modify code to the fly. When employed the right way, they Allow you to notice specifically how your code behaves during execution, that's a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-end developers. They assist you to inspect the DOM, check community requests, see true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can change disheartening UI concerns into workable responsibilities.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over working procedures and memory administration. Studying these equipment could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn out to be relaxed with Model Command systems like Git to comprehend code historical past, come across the precise instant bugs were introduced, and isolate problematic modifications.
In the end, mastering your equipment signifies likely beyond default settings and shortcuts — it’s about creating an intimate understanding of your advancement setting to ensure when difficulties occur, you’re not missing in the dead of night. The greater you realize your equipment, the more time you'll be able to devote solving the actual problem instead of fumbling through the process.
Reproduce the condition
One of the most significant — and infrequently forgotten — methods in powerful debugging is reproducing the trouble. Prior to jumping into your code or building guesses, developers require to create a dependable natural environment or situation exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug gets to be a activity of probability, typically leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as is possible. Request questions like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to be to isolate the precise situations less than which the bug happens.
As you’ve collected enough data, endeavor to recreate the trouble in your neighborhood atmosphere. This may imply inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, look at writing automated checks that replicate the edge situations or point out transitions involved. These exams don't just assist expose the situation but also avoid regressions Sooner or later.
Sometimes, The problem can be environment-certain — it would materialize only on particular working devices, browsers, or less than specific configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a attitude. It calls for endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you are presently halfway to repairing it. By using a reproducible circumstance, You should utilize your debugging applications extra effectively, test potential fixes safely, and communicate extra clearly together with your group or customers. It turns an abstract complaint right into a concrete obstacle — Which’s wherever builders prosper.
Examine and Realize the Error Messages
Error messages are often the most valuable clues a developer has when a little something goes Completely wrong. Rather then observing them as annoying interruptions, developers ought to learn to take care of mistake messages as direct communications in the procedure. They normally inform you just what happened, where by it took place, and often even why it happened — if you know the way to interpret them.
Start off by reading through the message thoroughly and in full. Lots of developers, especially when underneath time strain, look at the primary line and instantly get started generating assumptions. But deeper during the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines like google — examine and realize them first.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line number? What module or operate triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Understanding to acknowledge these can drastically accelerate your debugging system.
Some mistakes are obscure or generic, As well as in those circumstances, it’s vital to look at the context wherein the error occurred. Check out similar log entries, input values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings either. These typically precede larger sized problems and provide hints about likely bugs.
Finally, mistake messages are not your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint issues quicker, minimize debugging time, and become a far more successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When utilised proficiently, it offers authentic-time insights into how an software behaves, serving to you comprehend what’s happening under the hood without needing to pause execution or step through the code line by line.
A great logging technique starts with understanding what to log and at what degree. Frequent logging amounts contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for specific diagnostic facts through growth, Data for basic occasions (like successful start-ups), Alert for likely concerns that don’t break the applying, Mistake for real issues, and Lethal if the program can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and decelerate your program. Focus on critical functions, state improvements, input/output values, and important final decision points in the code.
Structure your log messages Plainly and regularly. Include things like context, for example timestamps, request IDs, and performance names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re In particular beneficial in generation environments exactly where stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a well-imagined-out logging solution, you are able to decrease the time it's going to take to spot concerns, attain deeper visibility into your programs, and Enhance the In general maintainability and reliability of one's code.
Assume Similar to a Detective
Debugging is not just a specialized process—it is a method of investigation. To successfully discover and deal with bugs, builders must method the method just like a detective solving a mystery. This attitude allows break down sophisticated challenges into manageable elements and comply with clues logically to uncover the foundation induce.
Begin by gathering evidence. Consider the indications of the problem: mistake messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, accumulate just as much relevant info as it is possible to with no leaping to conclusions. Use logs, examination situations, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Question by yourself: What may very well be leading to this conduct? Have any modifications lately been produced to the codebase? Has this issue happened in advance of underneath related conditions? The aim would be to narrow down alternatives and establish likely culprits.
Then, check your theories systematically. Attempt to recreate the condition in a very controlled atmosphere. For those who suspect a certain purpose or part, isolate it and verify if The difficulty persists. Like a detective conducting interviews, ask your code issues and Allow the results direct you closer to the reality.
Spend shut focus to small facts. Bugs usually disguise while in the least predicted places—just like a missing semicolon, an off-by-just one error, or maybe a race situation. Be complete and affected person, resisting the urge to patch The difficulty without having absolutely knowing it. Non permanent fixes could disguise the real challenge, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging approach can help you save time for potential challenges and assist Some others understand your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, tactic issues methodically, and grow to be more practical at uncovering concealed problems in intricate devices.
Write Exams
Composing assessments is among the most effective approaches to increase your debugging competencies and General advancement effectiveness. Assessments not simply help catch bugs early but additionally serve as a safety Internet that provides you self esteem when making modifications in your codebase. A very well-analyzed software is easier to debug because it permits you to pinpoint specifically the place and when a difficulty happens.
Begin with unit exams, which give attention to specific features or modules. These modest, isolated assessments can speedily expose irrespective of whether a selected bit of logic is Performing as predicted. Each time a examination fails, you right away know exactly where to appear, considerably decreasing some time used debugging. Device exams are Particularly helpful for catching regression bugs—issues that reappear just after Beforehand currently being mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These enable be certain that different parts of your software operate with each other effortlessly. They’re specially beneficial for catching bugs that happen in elaborate programs with numerous factors or companies interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Writing assessments also forces you to Assume critically about your code. To check a function thoroughly, you may need to understand its inputs, predicted outputs, and edge cases. This standard of comprehending Obviously prospects to higher code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug can be a strong starting point. Once the examination fails consistently, you'll be able to deal with fixing the bug and look at your exam pass when The problem is solved. This approach makes sure that the exact same bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing video game right into a structured and predictable procedure—supporting you capture extra bugs, quicker and even more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the challenge—observing your monitor for several hours, trying Remedy soon after Option. But One of the more underrated debugging tools is simply stepping away. Taking breaks can help you reset your intellect, cut down frustration, and often see the issue from the new standpoint.
If you're much too near the code for much too long, cognitive exhaustion sets in. You might start overlooking obvious faults or misreading code that you wrote just several hours before. With this condition, your brain turns into significantly less effective at issue-solving. A brief stroll, a coffee break, or even switching to a different endeavor for ten–15 minutes can refresh your concentrate. Numerous builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious perform within the background.
Breaks also assistance avert burnout, Specifically throughout longer debugging classes. Sitting in front of a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away allows you to return with renewed Electricity as well as a clearer way of thinking. You could possibly all of a sudden notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a superb rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that point to move all around, stretch, or do anything unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, nonetheless it really brings about faster and simpler debugging in the long run.
In a nutshell, having breaks just isn't an indication of weak spot—it’s a smart tactic. It gives your brain Place to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a thing valuable in the event you make time to mirror and assess what went Completely wrong.
Begin by asking oneself a number of critical thoughts as soon as the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit screening, code evaluations, or logging? The solutions usually reveal blind spots inside your workflow or comprehension and make it easier to Make more robust coding routines shifting forward.
Documenting bugs can also be an excellent habit. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring challenges or prevalent problems—which you could proactively stay away from.
In group environments, sharing what you've acquired from the bug using your peers can be Primarily highly effective. No matter whether it’s through a Slack information, a brief create-up, or A fast know-how-sharing session, aiding Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Discovering tradition.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your improvement journey. In fact, a number of the best developers are not the ones who generate best code, but those who continually learn from their problems.
In the end, Every single bug you fix adds a completely new layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Improving upon your debugging expertise usually takes time, get more info observe, and patience — even so the payoff is large. It tends to make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.